Using Gulp with Django: How to Improve Your Development Workflow
Discover how to increase your productivity as a web developer with Gulp and Django. Automate tasks like Sass compilation and CSS minification to save time and write better code. Follow the steps in this article to get started with Gulp and build great web applications.
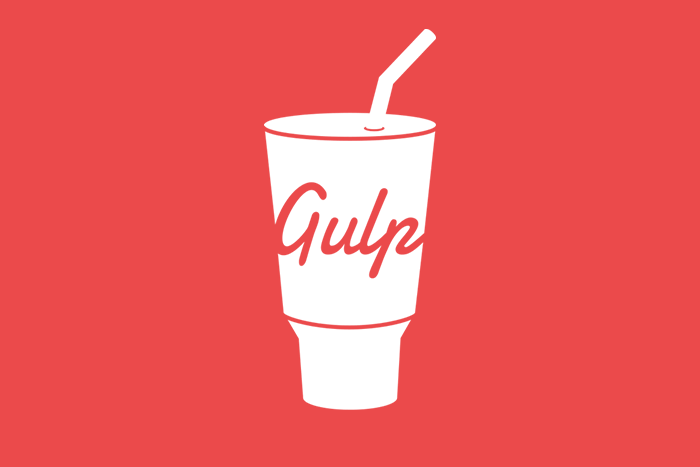
If you're a Django developer, you know how important it is to have a streamlined development workflow. Anything that can help you save time and improve your productivity is a welcome addition to your toolkit. That's where Gulp comes in.
Gulp is a popular task runner that helps automate repetitive tasks in your web development workflow. By using Gulp, you can easily perform tasks such as converting Sass to CSS, minifying CSS, and much more. And when you combine Gulp with Django, the benefits are even greater.
If you're not familiar with it, Django is a popular web framework that simplifies the process of building web applications. By integrating Gulp with Django, you can improve your development workflow and build better applications faster. In this post, we'll explore how to use Gulp with Django to increase your productivity and streamline your workflow.
In this post, I'll provide a step-by-step guide on how to use Gulp with Django to improve your development workflow. I'll start by explaining how to set up Gulp and create a Gulpfile.js in your Django project directory. Then, I'll show you how to use Gulp to convert Sass to CSS, minify CSS, and reload your HTML templates automatically.
By the end of this post, you'll have a clear understanding of how to integrate Gulp with Django and take advantage of the many benefits it offers. Whether you're a beginner or an experienced developer, you'll be able to use these techniques to streamline your development workflow and build better applications faster.
Section 1: Setting Up Gulp
Before you can start using Gulp with your Django project, you'll need to install Node.js on your machine. Here's how to do it:
- First, make sure you have Node.js installed on your machine. You can download it from the official Node.js website: https://nodejs.org/en/download/
- Next, navigate to your Django project directory in the terminal or command prompt and type the following command to install Gulp locally in your project:
$ npm install browser-sync del gulp gulp-autoprefixer gulp-clean-css gulp-cssbeautify gulp-file-include gulp-header gulp-htmlmin gulp-npm-dist gulp-plumber gulp-rename gulp-sass gulp-sourcemaps gulp-uglify gulp-wait merge-stream sass --save-dev
This will install Gulp locally in your Django project directory, where you can use it for your specific project.
That's it! You've now successfully installed Gulp in your Django project directory. In the next section, we'll create a Gulpfile.js in your Django project directory, which will define the tasks that Gulp will perform.
The first thing we need to do before start creating the Gulp tasks, we need to set up the Gulp environment. This involves importing the necessary modules and plugins, defining common file paths for the various source and output directories, and configuring the workflow for your specific needs.
In this code, we use the require()
method to import various modules and plugins that will be used in the Gulp tasks, such as gulp-autoprefixer
, browser-sync
, and gulp-clean-css
. These modules and plugins will help us to compile Sass files into CSS, add vendor prefixes, and minify our CSS files.
We also define the paths
object to provide common file paths for the various directories that we will be working with, such as the source and output directories for CSS files and third-party libraries.
const autoprefixer = require("gulp-autoprefixer");
const browserSync = require("browser-sync").create();
const cleanCss = require("gulp-clean-css");
const del = require("del");
const fs = require("fs");
const gulp = require("gulp");
const path = require("path");
const rename = require("gulp-rename");
const sass = require("gulp-sass")(require("sass"));
const sourcemaps = require("gulp-sourcemaps");
const spawn = require("child_process").spawn;
const wait = require("gulp-wait");
const { groupCollapsed } = require("console");
const paths = {
src: {
root: "./",
css: "./garupa_portal/static/assets/css",
scss: "./garupa_portal/static/assets/scss",
vendor: "./garupa_portal/static/assets/vendor",
},
};
Section 2: Converting Sass to CSS and Minifying CSS
One of the primary benefits of using Gulp with Django is the ability to automate repetitive tasks, such as converting Sass to CSS. Here's how to set up Gulp to perform this task:
- First, create a new file in your Django project directory called
Gulpfile.js
. This file will define the tasks that Gulp will perform. - In the
Gulpfile.js
file, require the necessary modules using the following code:
This code will require both Gulp and the gulp-sass
module, which will allow you to convert Sass to CSS.
Next, define a task to convert Sass to CSS using the following:
gulp.task("scss", function () {
return gulp
.src([
paths.src.scss + "/custom/**/*.scss",
paths.src.scss + "/portal/**/*.scss",
paths.src.scss + "/portal.scss",
])
.pipe(wait(500))
.pipe(sourcemaps.init())
.pipe(sass().on("error", sass.logError))
.pipe(
autoprefixer({
overrideBrowserslist: ["> 1%"],
})
)
.pipe(sourcemaps.write("."))
.pipe(gulp.dest(paths.src.css))
.pipe(browserSync.stream());
});
This code defines a Gulp task called scss
, which uses gulp.src()
to specify the location of your Sass files, gulp-sass
to convert the Sass to CSS, and gulp.dest()
to specify the location where the resulting CSS files should be saved.
Finally, run the scss
task by typing the following command in your terminal or command prompt:
$ gulp scss
This will run the scss
task and convert your Sass files to CSS.
That's it! You've now set up Gulp to convert Sass to CSS. In the next section, we'll show you how to use Gulp to minify your CSS files.
Section 3: Using Gulp to minify CSS
Minifying your CSS files can help to reduce their size and improve the performance of your web application. Here's how to set up Gulp to minify your CSS files:
- First, make sure you have completed the steps in the previous section to set up Gulp to convert Sass to CSS.
- In the
Gulpfile.js
file, add the following code to define a task to minify your CSS files:
gulp.task("minify:css", function () {
return gulp
.src([paths.src.css + "/portal.css"])
.pipe(cleanCss())
.pipe(
rename(function (path) {
// Updates the object in-place
path.extname = ".min.css";
})
)
.pipe(gulp.dest(paths.src.css));
});
This code defines a new Gulp task called minify:css
, which uses gulp.src()
to specify the location of your CSS files, gulp-clean-css
to minify the CSS, and gulp.dest()
to specify the location where the resulting minified CSS files should be saved.
To run the minify:css
task, type the following command in your terminal or command prompt:
$ gulp minify:css
This will run the minify:css
task and create minified versions of your CSS files.
That's it! You've now set up Gulp to minify your CSS files. In the next section, we'll show you how to use Gulp to reload your HTML templates automatically.
Section 4: Reloading HTML Templates
Reloading your HTML templates automatically can save you a lot of time during development, as you won't need to refresh your browser manually every time you make a change. Here's how to set up Gulp to do this for you:
- First, make sure you have completed the steps in the previous sections to set up Gulp to convert Sass to CSS and minify your CSS files.
- In the
Gulpfile.js
file, add the following code to define a task to reload your HTML templates automatically:
function reload(done) {
browserSync.reload();
done();
}
gulp.task("run:django", function (cb) {
const cmd = spawn("python", ["manage.py", "runserver", "0.0.0.0:8000"], {
stdio: "inherit",
});
cmd.on("close", function (code) {
console.log("Django application exited with code " + code);
cb(code);
});
});
gulp.task("browser:sync", function (cb) {
browserSync.init({
port: 3000,
proxy: "localhost:8000",
open: false,
reloadDelay: 300,
reloadDebounce: 500,
});
cb();
});
gulp.task("watch:files", function () {
gulp.watch(
[
`${paths.src.scss}/custom/**/*.scss`,
`${paths.src.scss}/portal/**/*.scss`,
`${paths.src.scss}/portal.scss`,
],
gulp.series("scss", "minify:css"),
reload
);
gulp.watch("./**/*.html", reload);
gulp.watch("./**/*.py", reload);
});
gulp.task("watch", gulp.parallel("run:django", "browser:sync", "watch:files"));
This code defines a new Gulp task called watch
, which uses the browser-sync
module to create a local development server that will automatically reload your HTML templates whenever changes are detected. The gulp.watch()
method is used to monitor changes in your CSS files and HTML templates, and the browserSync.reload
method is used to reload the browser when changes are detected.
Note that the proxy
option should be set to the address and port of your Django development server.
To run the browser-sync
task, type the following command in your terminal or command prompt:
$ gulp watch
As you can see, by using Gulp with Django, you can streamline your development workflow and automate repetitive tasks, such as converting Sass to CSS, minifying CSS files, and reloading your HTML templates automatically. This can help to save you time and improve your productivity when building web applications.
Some of the key benefits of using Gulp with Django include:
- Simplifying your development workflow by automating repetitive tasks
- Improving the performance of your web application by minifying your CSS files
- Enhancing the user experience during development by reloading your HTML templates automatically
If you're a web developer working with Django, we encourage you to try using Gulp to see how it can help you streamline your development workflow and build better web applications faster. With Gulp and Django, you can take your web development skills to the next level and create innovative and efficient solutions.
Bonus
If you want to further optimize your workflow when working with Gulp and Django, you might want to use the code below. This code includes additional tools to help manage third-party libraries and packages in your project, such as mapping library names to shorter, more concise names and moving files to specific subdirectories.
The code also includes a function to help clean up your project directory by deleting unnecessary files. If you want to take advantage of these tools, simply add the code to your project and modify it to fit your specific needs.
By using these additional tools, you can more effectively manage third-party dependencies and improve your workflow, making it easier and more efficient to build high-quality web applications with Gulp and Django.
const modules = {
"@popperjs/core": "popperjs",
bootstrap: "bootstrap",
chartist: "chartist",
"chartist-plugin-tooltips": "chartist-plugin-tooltips",
"flag-icons": "flag-icons",
jquery: "jquery",
leaflet: "leaflet",
notyf: "notyf",
nouislider: "nouislider",
onscreen: "onscreen",
simplebar: "simplebar",
"smooth-scroll": "smooth-scroll",
sweetalert2: "sweetalert2",
"vanillajs-datepicker": "vanillajs-datepicker",
vue: "vue",
axios: "axios"
};
function getModuleFilePaths(modules) {
return Object.keys(modules).map((module) => {
const moduleDir = path.join("./node_modules", module);
const vendorDir = path.join(paths.src.vendor, modules[module]);
return {
filePath: `${moduleDir}/**/*`,
directoryPath: vendorDir,
};
});
}
function moveFilesToDist(modulePath) {
const distPath = path.join(modulePath, "dist");
if (!fs.existsSync(distPath)) {
fs.mkdirSync(distPath, { recursive: true });
fs.readdir(modulePath, (err, files) => {
if (err) {
console.error("Error reading directory", err);
return;
}
files
.filter((file) => {
const filePath = path.join(modulePath, file);
const isDirectory = fs.lstatSync(filePath).isDirectory();
return !(isDirectory && file === "dist");
})
.forEach((file) => {
const oldPath = path.join(modulePath, file);
const newPath = path.join(distPath, file);
fs.renameSync(oldPath, newPath);
});
});
}
}
function createDelPatterns(dirs) {
return [
...dirs.map((dir) => `${dir}/**`),
...dirs.map((dir) => `!${dir}/dist`),
...dirs.map((dir) => `!${dir}/dist/**/*`),
];
}
Here's the entire package.json and Gulpfile.js that I have used in my Django project:
{
"name": "@alesimadev/django-bootstrap-dashboard",
"version": "1.0.0",
"description": "Dkjango Bootstrap Dashboard",
"main": "gulpfile.js",
"author": "Alex Silva",
"scripts": {
"build": "gulp",
"vendor": "gulp vendor",
"watch": "gulp watch"
},
"dependencies": {
"@popperjs/core": "^2.9.2",
"axios": "^1.3.4",
"bootstrap": "^5.2.3",
"chartist": "^0.11.4",
"chartist-plugin-tooltips": "^0.0.17",
"flag-icons": "^6.6.6",
"jquery": "^3.6.4",
"leaflet": "^1.9.3",
"notyf": "^3.10.0",
"nouislider": "^15.2.0",
"onscreen": "^1.4.0",
"simplebar": "^5.3.4",
"smooth-scroll": "^16.1.3",
"sweetalert2": "^11.0.18",
"vanillajs-datepicker": "^1.1.4",
"vue": "^3.2.45",
"waypoints": "^4.0.1"
},
"devDependencies": {
"browser-sync": "^2.27.4",
"del": "^6.0.0",
"gulp": "^4.0.2",
"gulp-autoprefixer": "^8.0.0",
"gulp-clean-css": "^4.3.0",
"gulp-cssbeautify": "^3.0.0",
"gulp-file-include": "^2.3.0",
"gulp-header": "^2.0.9",
"gulp-htmlmin": "^5.0.1",
"gulp-npm-dist": "^1.0.3",
"gulp-plumber": "^1.2.1",
"gulp-rename": "^2.0.0",
"gulp-sass": "^5.0.0",
"gulp-sourcemaps": "^3.0.0",
"gulp-uglify": "^3.0.2",
"gulp-wait": "^0.0.2",
"merge-stream": "^2.0.0",
"sass": "^1.56.2"
}
}
const autoprefixer = require("gulp-autoprefixer");
const browserSync = require("browser-sync").create();
const cleanCss = require("gulp-clean-css");
const del = require("del");
const fs = require("fs");
const gulp = require("gulp");
const path = require("path");
const rename = require("gulp-rename");
const sass = require("gulp-sass")(require("sass"));
const sourcemaps = require("gulp-sourcemaps");
const spawn = require("child_process").spawn;
const wait = require("gulp-wait");
const { groupCollapsed } = require("console");
const paths = {
src: {
root: "./",
css: "./garupa_portal/static/assets/css",
scss: "./garupa_portal/static/assets/scss",
vendor: "./garupa_portal/static/assets/vendor",
},
};
const modules = {
"@popperjs/core": "popperjs",
bootstrap: "bootstrap",
chartist: "chartist",
"chartist-plugin-tooltips": "chartist-plugin-tooltips",
"flag-icons": "flag-icons",
jquery: "jquery",
leaflet: "leaflet",
notyf: "notyf",
nouislider: "nouislider",
onscreen: "onscreen",
simplebar: "simplebar",
"smooth-scroll": "smooth-scroll",
sweetalert2: "sweetalert2",
"vanillajs-datepicker": "vanillajs-datepicker",
vue: "vue",
axios: "axios"
};
function reload(done) {
browserSync.reload();
done();
}
function getModuleFilePaths(modules) {
return Object.keys(modules).map((module) => {
const moduleDir = path.join("./node_modules", module);
const vendorDir = path.join(paths.src.vendor, modules[module]);
return {
filePath: `${moduleDir}/**/*`,
directoryPath: vendorDir,
};
});
}
function moveFilesToDist(modulePath) {
const distPath = path.join(modulePath, "dist");
if (!fs.existsSync(distPath)) {
fs.mkdirSync(distPath, { recursive: true });
fs.readdir(modulePath, (err, files) => {
if (err) {
console.error("Error reading directory", err);
return;
}
files
.filter((file) => {
const filePath = path.join(modulePath, file);
const isDirectory = fs.lstatSync(filePath).isDirectory();
return !(isDirectory && file === "dist");
})
.forEach((file) => {
const oldPath = path.join(modulePath, file);
const newPath = path.join(distPath, file);
fs.renameSync(oldPath, newPath);
});
});
}
}
function createDelPatterns(dirs) {
return [
...dirs.map((dir) => `${dir}/**`),
...dirs.map((dir) => `!${dir}/dist`),
...dirs.map((dir) => `!${dir}/dist/**/*`),
];
}
gulp.task("scss", function () {
return gulp
.src([
paths.src.scss + "/custom/**/*.scss",
paths.src.scss + "/portal/**/*.scss",
paths.src.scss + "/portal.scss",
])
.pipe(wait(500))
.pipe(sourcemaps.init())
.pipe(sass().on("error", sass.logError))
.pipe(
autoprefixer({
overrideBrowserslist: ["> 1%"],
})
)
.pipe(sourcemaps.write("."))
.pipe(gulp.dest(paths.src.css))
.pipe(browserSync.stream());
});
gulp.task("minify:css", function () {
return gulp
.src([paths.src.css + "/portal.css"])
.pipe(cleanCss())
.pipe(
rename(function (path) {
// Updates the object in-place
path.extname = ".min.css";
})
)
.pipe(gulp.dest(paths.src.css));
});
gulp.task("copy:vendor", function () {
return gulp
.src(getModuleFilePaths(modules).map((module) => module.filePath))
.pipe(
gulp.dest(function (file) {
for (const module in modules) {
if (file.path.includes(`${module}${path.sep}`)) {
const moduleName = modules[module];
const destPath = path.join(paths.src.vendor, moduleName);
if (!fs.existsSync(destPath)) {
fs.mkdirSync(destPath, { recursive: true });
}
return destPath;
}
}
return paths.src.vendor;
})
)
.on("end", function () {
getModuleFilePaths(modules).forEach((module) => {
moveFilesToDist(module.directoryPath);
});
});
});
gulp.task("clean:vendor", function () {
const moduleDirectories = getModuleFilePaths(modules).map(
(module) => module.directoryPath
);
const delPatterns = createDelPatterns(moduleDirectories);
return del(delPatterns, { force: true });
});
gulp.task("run:django", function (cb) {
const cmd = spawn("python", ["manage.py", "runserver", "0.0.0.0:8000"], {
stdio: "inherit",
});
cmd.on("close", function (code) {
console.log("Django application exited with code " + code);
cb(code);
});
});
gulp.task("browser:sync", function (cb) {
browserSync.init({
port: 3000,
proxy: "localhost:8000",
open: false,
reloadDelay: 300,
reloadDebounce: 500,
});
cb();
});
gulp.task("watch:files", function () {
gulp.watch(
[
`${paths.src.scss}/custom/**/*.scss`,
`${paths.src.scss}/portal/**/*.scss`,
`${paths.src.scss}/portal.scss`,
],
gulp.series("scss", "minify:css"),
reload
);
gulp.watch("./**/*.html", reload);
gulp.watch("./**/*.py", reload);
});
gulp.task("default", gulp.series("scss", "minify:css"));
gulp.task("watch", gulp.parallel("run:django", "browser:sync", "watch:files"));
gulp.task("vendor", gulp.series("copy:vendor", "clean:vendor"));
By using Gulp with Django, I've been able to significantly increase my productivity as a web developer. With Gulp, I can automate many of the repetitive tasks that used to take up a lot of my time, such as compiling Sass files, adding vendor prefixes, and minifying CSS files. This has freed up more time for me to focus on writing high-quality code and building great web applications.
Of course, every project is different, so the specific tasks and workflows that you'll want to automate with Gulp will depend on your individual needs and requirements. However, by following the steps outlined in this article, you'll have a solid foundation for setting up a Gulp environment in your Django project and automating many of the tasks that can slow down your workflow.
Whether you're an experienced web developer or just starting out, I encourage you to try using Gulp with Django and see how it can help you to work more efficiently and create higher-quality web applications. With the right tools and mindset, you can take your web development skills to the next level and build great things!