Simplify Static File Management in Django with Context Processors
Context processors can simplify referencing static files in Django templates. With a simple function that adds the static file path to the context dictionary, you can avoid hardcoding paths and save time and effort in the long run.
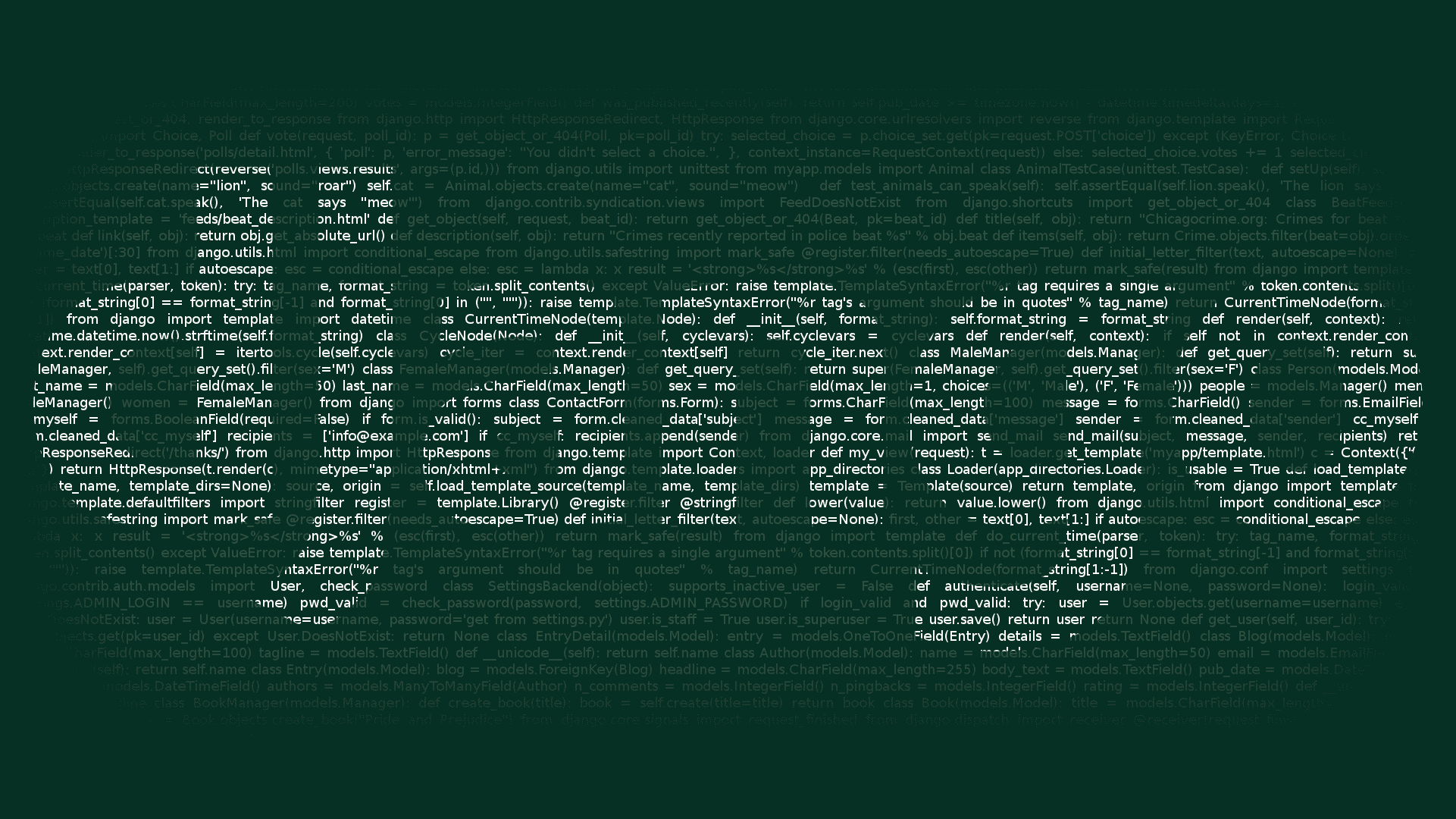
Are you tired of manually referencing the path of your static files in Django templates? Fortunately, there's an easy solution to this problem: context processors. In this article, we'll show you how to use context processors to simplify the process of managing static files in your Django project.
What are Context Processors?
In Django, context processors are functions that add data to the context dictionary for all templates in your project. This data can be anything that you want to make available in your templates, such as user data, global settings, or, in our case, the path of your static files.
Creating a Context Processor for Static Files
To create a context processor for your static files, you'll need to define a function that adds a new key-value pair to the context dictionary. The key should be a descriptive name for your static file path, such as ASSETS_ROOT
, and the value should be the path itself.
Here's an example of a simple context processor that adds the ASSETS_ROOT
key to the context dictionary:
from django.conf import settings
def assets_root(request):
return {'ASSETS_ROOT': settings.ASSETS_ROOT}
This context processor simply adds the ASSETS_ROOT
key to the context dictionary, with a value of settings.ASSETS_ROOT
. You'll need to define the ASSETS_ROOT
value in your settings.py
file. For example:
ASSETS_ROOT = os.path.join(BASE_DIR, 'static')
This code sets the ASSETS_ROOT
value to the path of your static
directory. You can customize this code to match the structure of your static files and project settings.
Using the Context Processor in Templates
Now that you've defined your context processor, you can use it in your templates to simplify the process of referencing your static files. Here's an example of how you can reference a stylesheet using the ASSETS_ROOT
context variable:
<link rel="stylesheet" type="text/css" href="{{ ASSETS_ROOT }}css/styles.css">
This code uses the ASSETS_ROOT
context variable to construct the URL for the styles.css
file in the css
directory of your static files. This approach eliminates the need to hardcode the path of your static files in your templates, making your code more maintainable and scalable.
Adding the Context Processor to Your Project
To add your context processor to your Django project, you'll need to update the TEMPLATES
setting in your settings.py
file. Here's an example of how you can add the assets_root
context processor to your project:
TEMPLATES = [
{
[...]
'OPTIONS': {
'context_processors': [
[...]
'yourapp.context_processors.assets_root', ],
},
},
]
This code adds the assets_root
context processor to the list of context processors for your project's templates. Now, whenever a template is rendered in your project, the ASSETS_ROOT
context variable will be available in the template context.
Conclusion
I hope this article has inspired you to use context processors to simplify the management of static files in your Django project. By adding a simple context processor that adds the static file path to the context dictionary, you can easily reference your static files in templates without hardcoding the path. This approach saves you time and effort in the long run, and makes your code more maintainable and scalable. So why not give context processors a try in your next Django project?